DataFrame in Script and Flow Showing Incorrect Column Order
I created a dataframe firstly with these 3 columns in this order: ["ID", "Amount", "Status"]
Then I reorder the column to ["Status", "ID", "Amount"]
However when shown in Windmill, it doesn't get reordered.
This is just a simplification of my code, in reality I'm working with more complex column management, but this issue makes me cannot proceed.
Here is the full code
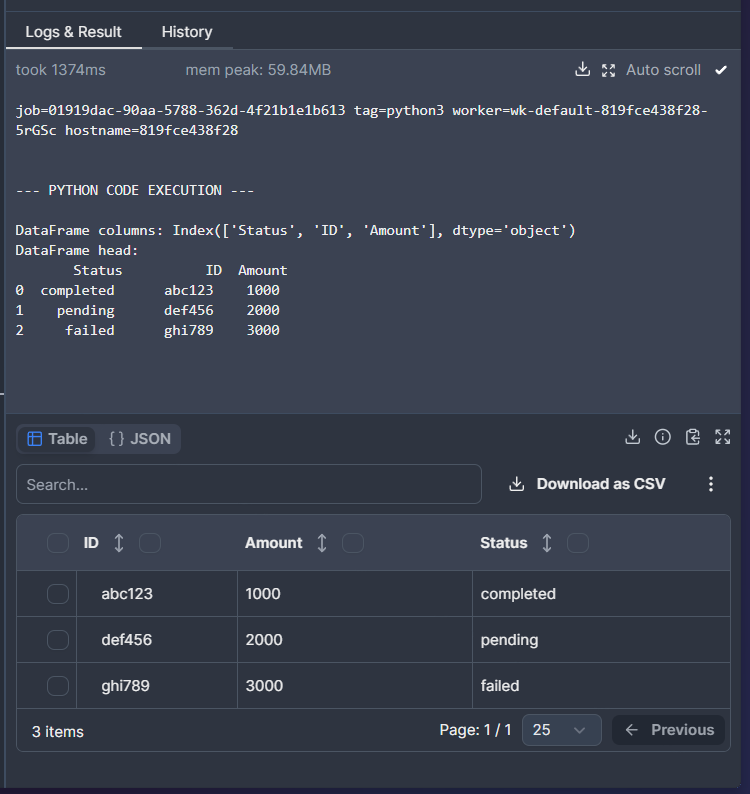
7 Replies
We store the result in postgresql JSONB which doesn't store the keys orders
fortunately there is a solution which is to add the columns in the right order at top
we could do that automatically but most people would expect the result to not have the headers as first row
can you please explain what you mean by
add the columns in the right order at top?
see second part of https://www.windmill.dev/docs/core_concepts/rich_display_rendering#table-row-object
Rich Display Rendering | Windmill
Windmill processes some outputs (from scripts or flows) intelligently to provide rich display rendering, allowing you to customize the display format of your results.
@rubenf sorry I don't really understand the docs.
I tried this but still doesn't work
Can you please help edit my code to follow your solution?
make sure the columns are at the top with the right order
println to make sure that's the case
if not, edit your code so that it is
I'm so sorry I'm so dumb... And I'm still a python beginner.
Can you please help to edit the code for me? I don't understand what you mean...
This code works. Hopefully it helps the others who has the same problem. Thanks
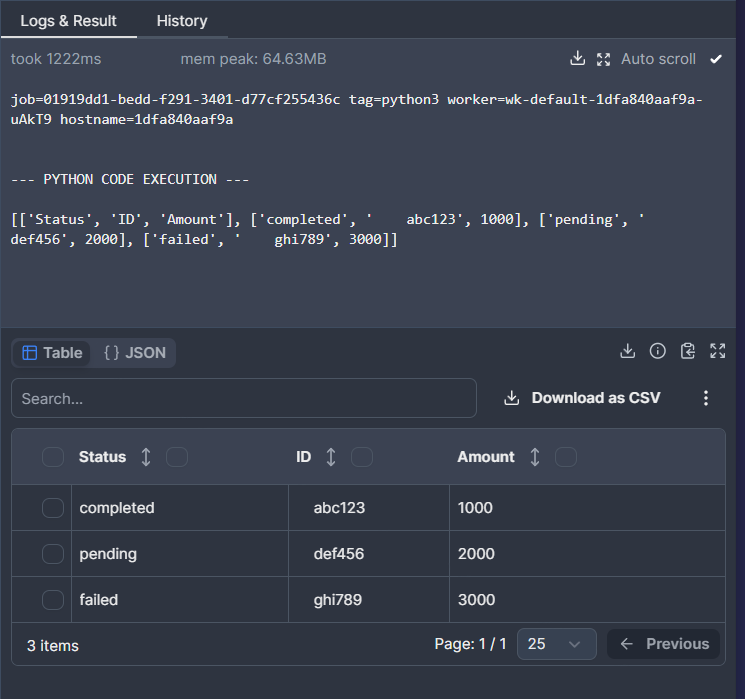