Weird script python problem
I have an interesting case: I have a python code that returns the transcript from an YouTube video. The transcript is returned if I run in my Windmill installed using docker-compose in my local machine - I used the commands from github. In the windmill SAAS it is not working - it shows the message that the video doesn't have the transcript (but it does). In my VPS installation is not working as well, showing the same error as the SAAS - I installed using the latest version today - 10/23/24
The pictures show
Python Code below in comment
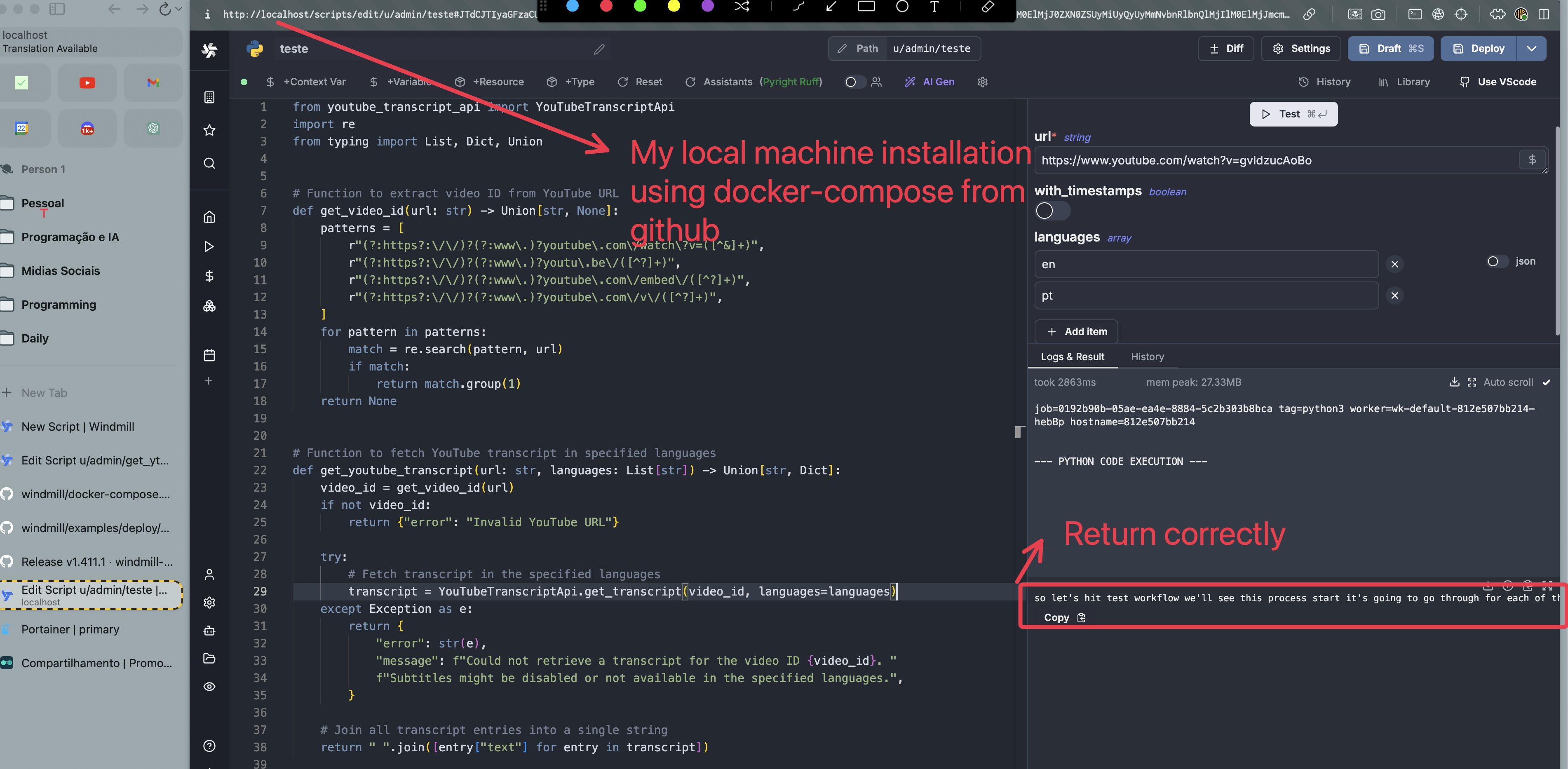
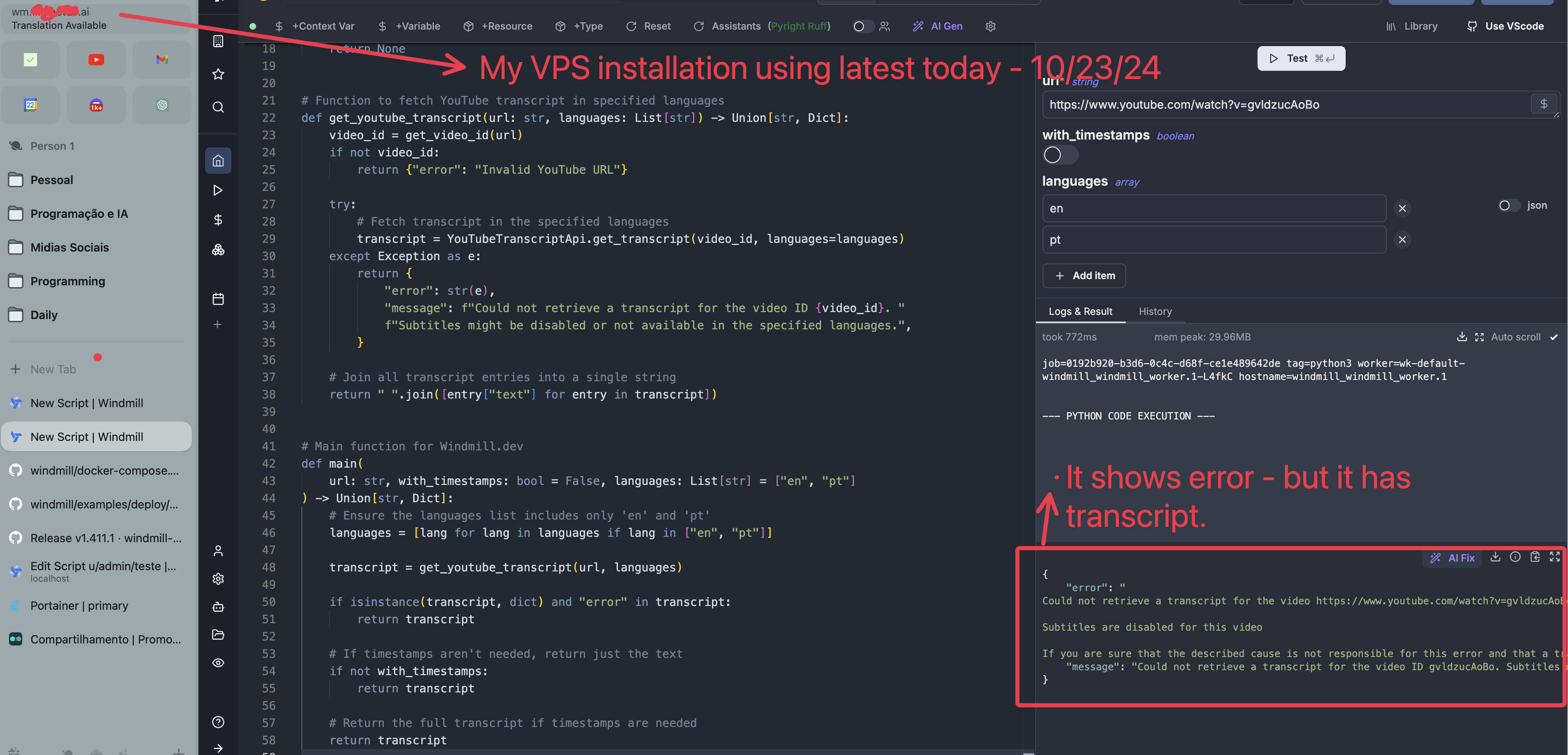
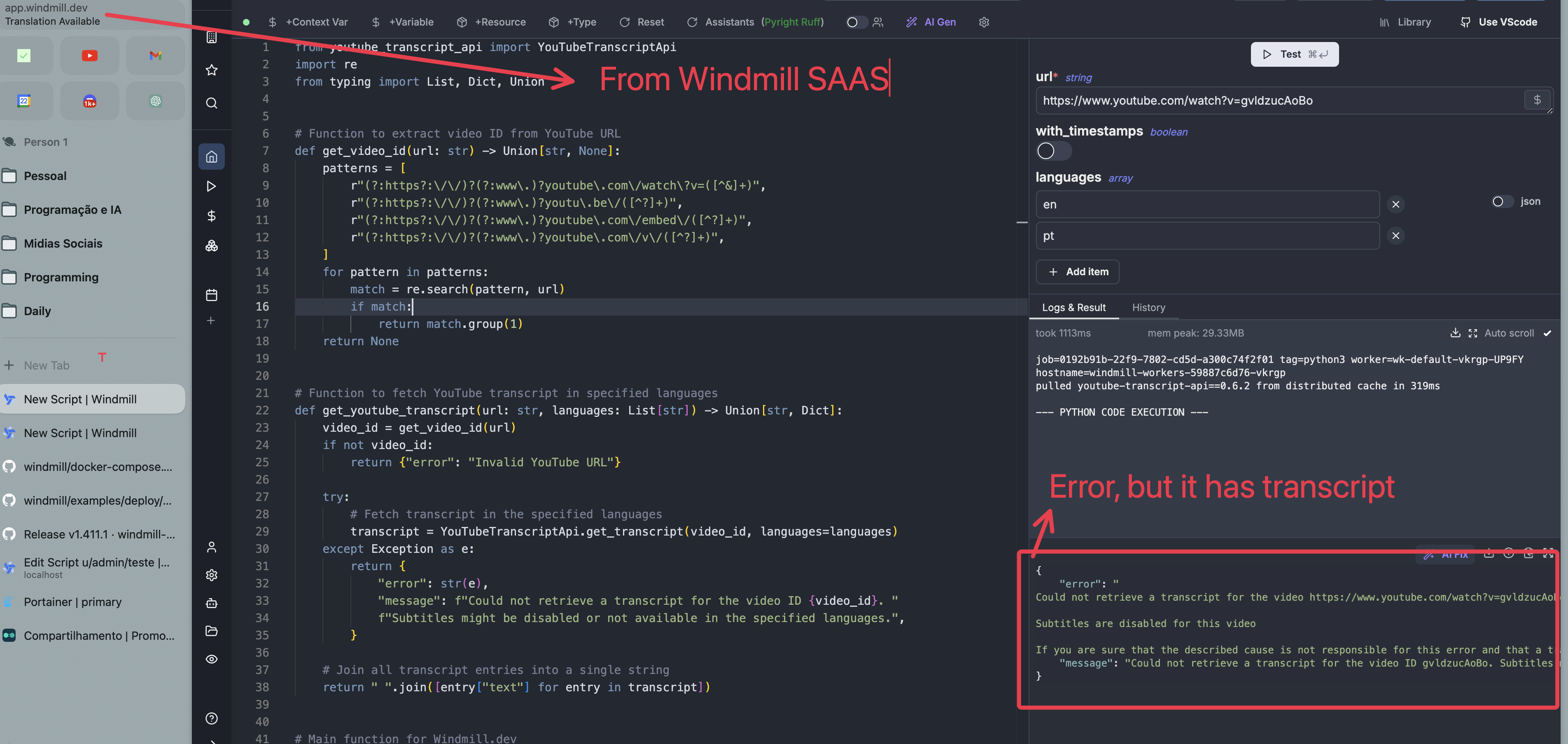
9 Replies
Python code:
Rest of the code:
I think it's youtube filtering those ips
basically google detecting that those ips are aws based and blocking them
Uhm. I didn’t think about it. I have a proxy server. I will try it and write back
@Elber Domingos if you solve this it would be great to know how im currently using an api service for this
lib version same and python version same? python sometimes make really weird things. what i see u dont use requirements nor extra_requirements...
i would always use extra_requirements and fix the lib version. just in case 😄
python from 3.8 to 3.12 has a lot of breaking changes including there libs
I am using Webshare to get a proxy, and the code is below. It worked, but sometimes it gets an IP that is blocked, and stop working. You could get a residencial proxy - more expensive - but it would work probably always.
Code for transcript with proxy
@Elber DomingosI'm just using RapidAPI now. Some providers give you 500 transcripts per day for free, and it's working always.And if YouTube has no transcript, Rapid API also has an MP3 converter which you could then use for transcriptions.
that is cool. Thank you - I will use it for sure